kuligs2 Thanks for your reply, however I'm not sure if I was clear enough on what I have and what I'm looking to do.
Here's my Player Script:
`extends CharacterBody3D
Variables
const SPEED = 7
const JUMP_VELOCITY = 7
var acceleration: float = 4.0
const LERP_VAL: float = 0.15
var gamepadSensitivity: float = 0.04
var gravity = ProjectSettings.get_setting("physics/3d/default_gravity")
@onready var spring_arm_pivot = $SpringArmPivot
@onready var spring_arm_3d = $SpringArmPivot/SpringArm3D
@onready var Armature = $Armature
@onready var animation_tree = $AnimationTree
@onready var animation_state = $AnimationTree.get("parameters/playback")
Quit Game Window
func _unhandled_input(event):
if Input.is_action_just_pressed("Quit"):
get_tree().quit()
Start Method
func _ready():
pass
#Input.set_mouse_mode(Input.MOUSE_MODE_CAPTURED) #Disable mouse on screen
Main Method
func _physics_process(delta):
#Rotate Camera Horizontal
spring_arm_pivot.rotate_y(Input.get_action_strength("Rotate_Right") * gamepadSensitivity * -1)
spring_arm_pivot.rotate_y(Input.get_action_strength("Rotate_Left") * gamepadSensitivity)
#Rotate Camera Vertical
spring_arm_3d.rotate_x(Input.get_action_strength("Rotate_Down") * gamepadSensitivity * -1)
spring_arm_3d.rotate_x(Input.get_action_strength("Rotate_Up") * gamepadSensitivity)
spring_arm_3d.rotation.x = clamp(spring_arm_3d.rotation.x, deg_to_rad(-50), deg_to_rad(30)) #Clamp Rotation
# Add the gravity.
if not is_on_floor():
velocity.y -= gravity * delta
# Handle Jump.
if Input.is_action_just_pressed("Jump") and is_on_floor():
velocity.y = JUMP_VELOCITY
#Player Movement
var input_dir = Input.get_vector("Move_Left", "Move_Right", "Move_Forward", "Move_Back")
var direction = (transform.basis * Vector3(input_dir.x, 0, input_dir.y)).normalized()
direction = direction.rotated(Vector3.UP, spring_arm_pivot.rotation.y) #Player Direction to Camera
if direction:
velocity.x = lerp(velocity.x, direction.x * SPEED, LERP_VAL)
velocity.z = lerp(velocity.z, direction.z * SPEED, LERP_VAL)
Armature.rotation.y = lerp_angle(Armature.rotation.y, atan2(-velocity.x, -velocity.z), LERP_VAL) #Rotate Player In direction moving
else:
velocity.x = lerp(velocity.x, 0.0 * SPEED, LERP_VAL)
velocity.z = lerp(velocity.z, 0.0 * SPEED, LERP_VAL)
#Play Animations
animation_tree.set("parameters/BlendSpace1D/blend_position", velocity.length() / SPEED)
move_and_slide()
`
This is a 3D Platformer controller using Gamepad. The Camera rotates around the player and doesn't effect it's direction, The Player walks in any direction, inlcuding towards you - similar to Mario64/Simpsons Game etc.
Here's my AnimationTree3D which is only populated with the one animation Blendspace1D (Idle/Walk/Run), I will add the other animations later.

Here's my BlendSpace1D, The first Value at '0' is Idle, in the middle is Walk and the last at '1' is Run.
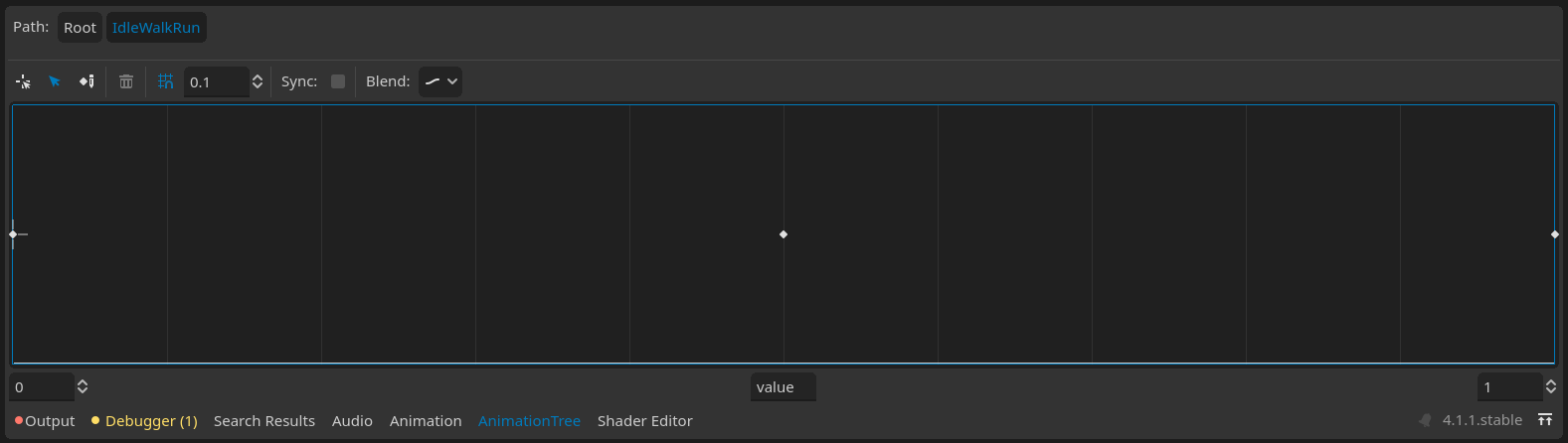
For clarification, here's my Player's node tree.
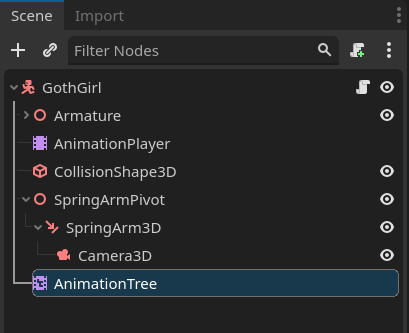
In the BlendSpace1D, I don't know whether I need to change the Values, it starts at '0' and ends at '1'. Do I need '0' to '2'?
In my script, how do I trigger all the animations in the BlendSpace1D to smoothly animate from Idle > Walk > Run?
Will having acceleration in my Player's movement fix this, or am I missing something? Currentlt my Player's speed is a constant and is fixed.
I've used:
animation_tree.set("parameters/BlendSpace1D/blend_position", velocity.length() / SPEED)
But this doesn't animate the Player from Idle/Walk/Run. All I've been able to do so far is get Idle > Run, or Idle > Walk, not all three animations.
I'm sure I could set up some variables to trigger the specific animations of Idle, Walk, Run when the Player reaches certain speeds, but this sounds counterintuitive when I think that's what the BlendSpace1D is meant for - correct me if i'm wrong.
Thanks.